armi.reactor.converters.uniformMesh module
Converts reactor with arbitrary axial meshing (e.g. multiple assemblies with different axial meshes) to one with a global uniform axial mesh.
Useful for preparing inputs for physics codes that require structured meshes from a more flexible ARMI reactor mesh.
This is implemented generically but includes a concrete subclass for neutronics-specific parameters. This is used for build input files for codes like DIF3D which require axially uniform meshes.
Requirements
Build an average reactor with aligned axial meshes from a reactor with arbitrarily unaligned axial meshes in a way that conserves nuclide mass
Translate state information computed on the uniform mesh back to the unaligned mesh.
For neutronics cases, all neutronics-related block params should be translated, as well as the multigroup real and adjoint flux.
Warning
This procedure can cause numerical diffusion in some cases. For example, if a control rod tip block has a large coolant block below it, things like peak absorption rate can get lost into it. We recalculate some but not all reaction rates in the re-mapping process based on a flux remapping. To avoid this, finer meshes will help. Always perform mesh sensitivity studies to ensure appropriate convergence for your needs.
Examples
converter = uniformMesh.NeutronicsUniformMeshConverter() converter.convert(reactor) uniformReactor = converter.convReactor # do calcs, then: converter.applyStateToOriginal()
The mesh mapping happens as described in the figure:
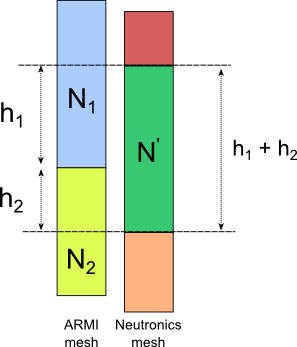
- class armi.reactor.converters.uniformMesh.UniformMeshGenerator(r, minimumMeshSize=None)[source]
Bases:
object
This class generates a common axial mesh to for the uniform mesh converter to use. The generation algorithm starts with the simple
average1DWithinTolerance
utility function to compute a representative “average” of the assembly meshes in the reactor. It then modifies that mesh to more faithfully represent important material boundaries of fuel and control absorber material.The decusping feature is controlled with the case setting
uniformMeshMinimumSize
. If no value is provided for this setting, the uniform mesh generator will skip the decusping step and just provide the result of_computeAverageAxialMesh
.Initialize an object to generate an appropriate common axial mesh to use for uniform mesh conversion.
- Parameters:
- generateCommonMesh()[source]
Generate a common axial mesh to use.
A core-wide mesh is computed via
_computeAverageAxialMesh
which operates by first collecting all the mesh points for every assembly (allMeshes
) and then averaging them together usingaverage1DWithinTolerance
. An attempt to preserve fuel and control material boundaries is accomplished by moving fuel region boundaries to accomodate control rod boundaries. Note this behavior only occurs by calling_decuspAxialMesh
which is dependent onminimumMeshSize
being defined (this is controlled by theuniformMeshMinimumSize
setting).Implementation: Produce a mesh with a size no smaller than a user-specified value. I_ARMI_UMC_MIN_MESHIf a minimum mesh size
minimumMeshSize
is provided, calls_decuspAxialMesh
on the core-wide mesh to maintain that minimum size while still attempting to honor fuel and control material boundaries. Relies ultimately on_filterMesh
to remove mesh points that violate the minimum size. Note that_filterMesh
will always respect the minimum mesh size, even if this means losing a mesh point that represents a fuel or control material boundary.Notes
Attempts to reduce the effect of fuel and control rod absorber smearing (“cusping” effect) by keeping important material boundaries in the common mesh.
- class armi.reactor.converters.uniformMesh.UniformMeshGeometryConverter(cs=None)[source]
Bases:
GeometryConverter
This geometry converter can be used to change the axial mesh structure of the reactor core.
Notes
There are several staticmethods available on this class that allow for:
Creation of a new reactor without applying a new uniform axial mesh. See: <UniformMeshGeometryConverter.initNewReactor>
Creation of a new assembly with a new axial mesh applied. See: <UniformMeshGeometryConverter.makeAssemWithUniformMesh>
Resetting the parameter state of an assembly back to the defaults for the provided block parameters. See: <UniformMeshGeometryConverter.clearStateOnAssemblies>
Mapping number densities and block parameters between one assembly to another. See: <UniformMeshGeometryConverter.setAssemblyStateFromOverlaps>
This class is meant to be extended for specific physics calculations that require a uniform mesh. The child types of this class should define custom reactorParamsToMap and blockParamsToMap attributes, and the _setParamsToUpdate method to specify the precise parameters that need to be mapped in each direction between the non-uniform and uniform mesh assemblies. The definitions should avoid mapping block parameters in both directions because the mapping process will cause numerical diffusion. The behavior of setAssemblyStateFromOverlaps is dependent on the direction in which the mapping is being applied to prevent the numerical diffusion problem.
“in” is used when mapping parameters into the uniform assembly from the non-uniform assembly.
“out” is used when mapping parameters from the uniform assembly back to the non-uniform assembly.
Warning
If a parameter is calculated by a physics solver while the reactor is in its converted (uniform mesh) state, that parameter must be included in the list of reactorParamNames or blockParamNames to be mapped back to the non-uniform reactor; otherwise, it will be lost. These lists are defined through the _setParamsToUpdate method, which uses the reactorParamMappingCategories and blockParamMappingCategories attributes and applies custom logic to create a list of parameters to be mapped in each direction.
- reactorParamMappingCategories = {'in': [], 'out': []}
- blockParamMappingCategories = {'in': [], 'out': []}
- convert(r=None)[source]
Create a new reactor core with a uniform mesh.
Given a source Reactor,
r
, as input and when_hasNonUniformAssems
isFalse
, a new Reactor is created ininitNewReactor
. This new Reactor contains copies of select information from the input source Reactor (e.g., Operator, Blueprints, cycle, timeNode, etc). The uniform mesh to be applied to the new Reactor is calculated in_generateUniformMesh
(see I_ARMI_UMC_NON_UNIFORM and I_ARMI_UMC_MIN_MESH). New assemblies with this uniform mesh are created in_buildAllUniformAssemblies
and added to the new Reactor. Core-level parameters are then mapped from the source Reactor to the new Reactor in_mapStateFromReactorToOther
. Finally, the core-wide axial mesh is updated on the new Reactor viaupdateAxialMesh
.Implementation: Map select parameters from composites on the original mesh to the new mesh. I_ARMI_UMC_PARAM_FORWARDIn
_mapStateFromReactorToOther
, Core-level parameters are mapped from the source Reactor to the new Reactor. If requested, block-level parameters can be mapped using an averaging equation as described insetAssemblyStateFromOverlaps
.
- static initNewReactor(sourceReactor, cs)[source]
Build a new, yet empty, reactor with the same settings as sourceReactor.
- Parameters:
sourceReactor (
Reactor
) – original reactor object to be copiedcs (Setting) – Complete settings object
- applyStateToOriginal()[source]
Apply the state of the converted reactor back to the original reactor, mapping number densities and block parameters.
Implementation: Map select parameters from composites on the new mesh to the original mesh. I_ARMI_UMC_PARAM_BACKWARDTo ensure that the parameters on the original Reactor are from the converted Reactor, the first step is to clear the Reactor-level parameters on the original Reactor (see
_clearStateOnReactor
)._mapStateFromReactorToOther
is then called to map Core-level parameters and, optionally, averaged Block-level parameters (see I_ARMI_UMC_PARAM_FORWARD).
- static makeAssemWithUniformMesh(sourceAssem, newMesh, paramMapper=None, mapNumberDensities=True, includePinCoordinates=False)[source]
Build new assembly based on a source assembly but apply the uniform mesh.
Notes
This creates a new assembly based on the provided source assembly, applies a new uniform mesh and then maps number densities and block-level parameters to the new assembly from the source assembly.
- Parameters:
sourceAssem (Assembly <armi.reactor.assemblies.Assembly> object) – Assembly that is used to map number densities and block-level parameters to a new mesh structure.
newMesh (List[float]) – A list of the new axial mesh coordinates of the blocks. Note that these mesh coordinates are in cm and should represent the top axial mesh coordinates of the new blocks.
paramMapper (ParamMapper) – Object that contains list of parameters to be mapped and has methods for mapping
mapNumberDensities (bool, optional) – If True, number densities will be mapped from the source assembly to the new assembly. This is True by default, but this can be set to False to only map block-level parameters if the names are provided in blockParamNames. It can be useful to set this to False in circumstances where the
setNumberDensitiesFromOverlaps
does not conserve mass and for some edge cases. This can show up in specific instances with moving meshes (i.e., control rods) in some applications. In those cases, the mapping of number densities can be treated independent of this more general implementation.
See also
setAssemblyStateFromOverlaps
This can be used to reverse the number density and parameter mappings between two assemblies.
- static setAssemblyStateFromOverlaps(sourceAssembly, destinationAssembly, paramMapper, mapNumberDensities=False, calcReactionRates=False)[source]
Set state data (i.e., number densities and block-level parameters) on a assembly based on a source assembly with a different axial mesh.
This solves an averaging equation from the source to the destination.
\[<P> = \frac{\int_{z_1}^{z_2} P(z) dz}{\int_{z_1}^{z_2} dz}\]which can be solved piecewise for z-coordinates along the source blocks.
Notes
If the parameter is volume integrated (e.g., flux, linear power) then calculate the fractional contribution from the source block.
If the parameter is not volume integrated (e.g., volumetric reaction rate) then calculate the fraction contribution on the destination block. This smears the parameter over the destination block.
- Parameters:
sourceAssembly (Assembly) – assem that has the state
destinationAssembly (Assembly) – assem that has is getting the state from sourceAssembly
paramMapper (ParamMapper) – Object that contains list of parameters to be mapped and has methods for mapping
mapNumberDensities (bool, optional) – If True, number densities will be mapped from the source assembly to the destination assembly. This is True by default, but this can be set to False to only map block-level parameters if the names are provided in blockParamNames. It can be useful to set this to False in circumstances where the
setNumberDensitiesFromOverlaps
does not conserve mass and for some edge cases. This can show up in specific instances with moving meshes (i.e., control rods) in some applications. In those cases, the mapping of number densities can be treated independent of this more general implementation.calcReactionRates (bool, optional) – If True, the neutron reaction rates will be calculated on each block within the destination assembly. Note that this will skip the reaction rate calculations for a block if it does not contain a valid multi-group flux.
See also
setNumberDensitiesFromOverlaps
does this but does smarter caching for number densities.
- clearStateOnAssemblies(blockParamNames=None, cache=True)[source]
Clears the parameter state of blocks for a list of assemblies.
- Parameters:
assems (List[Assembly <armi.reactor.assemblies.Assembly>]) – List of assembly objects.
blockParamNames (List[str], optional) – A list of block parameter names to clear on the given assemblies.
cache (bool) – If True, the block parameters that were cleared are stored and returned as a dictionary of
{b: {param1: val1, param2: val2}, b2: {...}, ...}
- updateReactionRates()[source]
Update reaction rates on converted assemblies.
Notes
In some cases, we may want to read flux into a converted reactor from a pre-existing physics output instead of mapping it in from the pre-conversion source reactor. This method can be called after reading that flux in to calculate updated reaction rates derived from that flux.
- class armi.reactor.converters.uniformMesh.NeutronicsUniformMeshConverter(cs=None, calcReactionRates=True)[source]
Bases:
UniformMeshGeometryConverter
A uniform mesh converter that specifically maps neutronics parameters.
Notes
This uniform mesh converter is intended for setting up an eigenvalue (fission-source) neutronics solve. There are no block parameters that need to be mapped in for a basic eigenvalue calculation, just number densities. The results of the calculation are mapped out (i.e., back to the non-uniform mesh). The results mapped out include things like flux, power, and reaction rates.
Warning
If a parameter is calculated by a physics solver while the reactor is in its converted (uniform mesh) state, that parameter must be included in the list of reactorParamNames or blockParamNames to be mapped back to the non-uniform reactor; otherwise, it will be lost. These lists are defined through the _setParamsToUpdate method, which uses the reactorParamMappingCategories and blockParamMappingCategories attributes and applies custom logic to create a list of parameters to be mapped in each direction.
- Parameters:
cs (obj, optional) – Case settings object.
calcReactionRates (bool, optional) – Set to True by default, but if set to False the reaction rate calculation after the neutron flux is remapped will not be calculated.
- reactorParamMappingCategories = {'in': ['neutronics'], 'out': ['neutronics']}
- blockParamMappingCategories = {'in': [], 'out': ['detailedAxialExpansion', 'multi-group quantities', 'pinQuantities']}
- class armi.reactor.converters.uniformMesh.GammaUniformMeshConverter(cs=None)[source]
Bases:
UniformMeshGeometryConverter
A uniform mesh converter that specifically maps gamma parameters.
Notes
This uniform mesh converter is intended for setting up a fixed-source gamma transport solve. Some block parameters from the neutronics solve, such as b.p.mgFlux, may need to be mapped into the uniform mesh reactor so that the gamma source can be calculated by the ARMI plugin performing gamma transport. Parameters that are updated with gamma transport results, such as powerGenerated, powerNeutron, and powerGamma, need to be mapped back to the non-uniform reactor.
Warning
If a parameter is calculated by a physics solver while the reactor is in its converted (uniform mesh) state, that parameter must be included in the list of reactorParamNames or blockParamNames to be mapped back to the non-uniform reactor; otherwise, it will be lost. These lists are defined through the _setParamsToUpdate method, which uses the reactorParamMappingCategories and blockParamMappingCategories attributes and applies custom logic to create a list of parameters to be mapped in each direction.
- reactorParamMappingCategories = {'in': ['neutronics'], 'out': ['neutronics']}
- blockParamMappingCategories = {'in': ['multi-group quantities'], 'out': ['gamma', 'neutronics']}
- class armi.reactor.converters.uniformMesh.ParamMapper(reactorParamNames, blockParamNames, b)[source]
Bases:
object
Utility for parameter setters/getters that can be used when transferring data from one assembly to another during the mesh conversion process. Stores some data like parameter defaults and properties to save effort of accessing paramDefs many times for the same data.
Initialize the list of parameter defaults.
The ParameterDefinitionCollection lookup is very slow, so this we do it once and store it as a hashed list.
- armi.reactor.converters.uniformMesh.setNumberDensitiesFromOverlaps(block, overlappingBlockInfo)[source]
Set number densities on a block based on overlapping blocks.
A conservation of number of atoms technique is used to map the non-uniform number densities onto the uniform neutronics mesh. When the number density of a height \(H\) neutronics mesh block \(N^{\prime}\) is being computed from one or more blocks in the ARMI mesh with number densities \(N_i\) and heights \(h_i\), the following formula is used:
\[N^{\prime} = \sum_i N_i \frac{h_i}{H}\]