Note
Go to the end to download the full example code
Make a Cartesian grid.
This builds a Cartesian grid with squares 1 cm square, with the z-coordinates provided explicitly. It is also offset in 3D space to X, Y, Z = 10, 5, 5 cm.
Learn more about grids
.
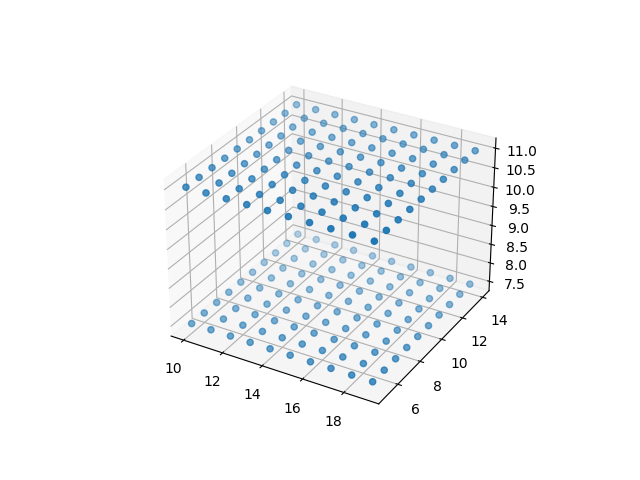
import itertools
import matplotlib.pyplot as plt
from armi import configure
from armi.reactor import grids
configure(permissive=True)
fig = plt.figure()
zCoords = [1, 4, 8]
cartesian_grid = grids.CartesianGrid(
unitSteps=((1, 0), (0, 1)),
bounds=(None, None, zCoords),
offset=(10, 5, 5),
)
xyz = []
# the grid is infinite in i and j so we will just plot the first 10 items
for i, j, k in itertools.product(range(10), range(10), range(len(zCoords) - 1)):
xyz.append(cartesian_grid[i, j, k].getGlobalCoordinates())
ax = fig.add_subplot(1, 1, 1, projection="3d")
x, y, z = zip(*xyz)
ax.scatter(x, y, z)
plt.show()
Total running time of the script: ( 0 minutes 0.079 seconds)