armi.reactor.converters.uniformMesh module¶
Converts reactor with arbitrary axial meshing (e.g. multiple assemblies with different axial meshes) to one with a global uniform axial mesh.
Useful for preparing inputs for physics codes that require structured meshes from a more flexible ARMI reactor mesh.
This is implemented generically but includes a concrete subclass for neutronics-specific parameters. This is used for build input files for codes like DIF3D which require axially uniform meshes.
Requirements¶
Build an average reactor with aligned axial meshes from a reactor with arbitrarily unaligned axial meshes in a way that conserves nuclide mass
Translate state information computed on the uniform mesh back to the unaligned mesh.
For neutronics cases, all neutronics-related block params should be translated, as well as the multigroup real and adjoint flux.
Examples
converter = uniformMesh.NeutronicsUniformMeshConverter() uniformReactor = converter.convert(reactor) # do calcs, then: converter.applyStateToOriginal()
The mesh mapping happens as described in the figure:
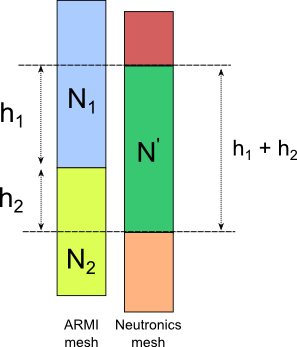
-
class
armi.reactor.converters.uniformMesh.
UniformMeshGeometryConverter
(cs=None)[source]¶ Bases:
armi.reactor.converters.geometryConverters.GeometryConverter
Build uniform mesh version of the source reactor
-
static
makeAssemWithUniformMesh
(sourceAssem, newMesh)[source]¶ Build new assembly based on a source assembly but apply the uniform mesh.
The new assemblies must have appropriately mapped number densities as input for a neutronics solve. They must also have other relevant state parameters for follow-on steps. Thus, this maps many parameters from the ARMI mesh to the uniform mesh.
See also
applyStateToOriginal()
basically the reverse on the way out.
-
_buildAllUniformAssemblies
()[source]¶ Loop through each new block for each mesh point and apply conservation of atoms.
We use the submesh and allow blocks to be as small as the smallest submesh to avoid unnecessarily diffusing small blocks into huge ones (e.g. control blocks into plenum).
-
_clearStateOnReactor
(reactor)[source]¶ Delete existing state that will be updated so they don’t increment.
The summations should start at zero but will happen for all overlaps.
-
static
-
class
armi.reactor.converters.uniformMesh.
NeutronicsUniformMeshConverter
(cs=None, calcReactionRates=True)[source]¶ Bases:
armi.reactor.converters.uniformMesh.UniformMeshGeometryConverter
A uniform mesh converter that specifically maps neutronics parameters.
This is useful for codes like DIF3D.
Notes
If a case runs where two mesh conversions happen one after the other (e.g. a fixed source gamma transport step that needs appropriate fission rates), it is essential that the neutronics params be mapped onto the newly converted reactor as well as off of it back to the source reactor.
- Parameters
cs (obj, optional) – Case settings object.
calcReactionRates (bool, optional) – Set to True by default, but if set to False the reaction rate calculation after the neutron flux is remapped will not be calculated.
-
armi.reactor.converters.uniformMesh.
_setNumberDensitiesFromOverlaps
(block, overlappingBlockInfo)[source]¶ Set number densities on a block based on overlapping blocks
A conservation of number of atoms technique is used to map the non-uniform number densities onto the uniform neutronics mesh. When the number density of a height \(H\) neutronics mesh block \(N^{\prime}\) is being computed from one or more blocks in the ARMI mesh with number densities \(N_i\) and heights \(h_i\), the following formula is used:
\[N^{\prime} = \sum_i N_i \frac{h_i}{H}\]See also
_setStateFromOverlaps()
does this for state other than number densities.
-
armi.reactor.converters.uniformMesh.
_setStateFromOverlaps
(sourceAssembly, destinationAssembly, setter, getter, paramNames=None)[source]¶ Set state info on a assembly based on a source assembly with a different axial mesh
This solves an averaging equation from the source to the destination.
\[<P> = \frac{\int_{z_1}^{z_2} P(z) dz}{\int_{z_1}^{z_2} dz}\]which can be solved piecewise for z-coordinates along the source blocks.
For volume-integrated params (like block power), one must note that the piecewise integrals have the fraction of overlapping source height in them, not the overlapping height itself.
- Parameters
sourceAssembly (Assembly) – assem that has the state
destinationAssembly (Assembly) – assem that has is getting the state from sourceAssembly
setter (function) – A function that takes (block, val) and sets val as state on block.
getter (function) – takes block as an argument, returns relevant state that has __add__ capabilities.
paramNames (list) – List of param names to set/get. Ok to skip if getter does not use param names.
Notes
setter and getter are meant to be generated with particular state info (e.g. mgFlux or params).
See also
_setNumberDensitiesFromOverlaps()
does this but does smarter caching for number densities.