10.2.5. armicontrib.dif3d.inputWriters module¶
Components for writing DIF3D inputs from ARMI models.
DIF3D Inputs can be specified in a combination of binary interface files (see, e.g.,
armi.nuclearDataIO
) and/or textual input in free format (order matters)
or fixed format (column number matters).
DIF3D will convert any input into binary interface files on initialization, so there may be some performance advantage of using binary when possible. However, the implementation currently produces text-based input files. These are created using the Jinja2 template engine, which aims to separate boilerplate text from case-dependent contents through text interpolation.
Geometry details are specified on what DIF3D calls the A.NIP3 cards. To support
different geometries (Hex, Cartesian, Triangular, RZ, etc.) we support subclassing of
the GeometryWriter
subclasses, which are composed into Dif3dWriter
objects.
Ultimately, these data, along with material information, execution settings, etc. are
packaged in a payload dictionary in Dif3dWriter._buildTemplateData()
, then
passed to the Jinja2 template to generate the actual DIF3D input file. The template file
itself can be found at armicontrib/dif3d/templates/dif3d.inp
.
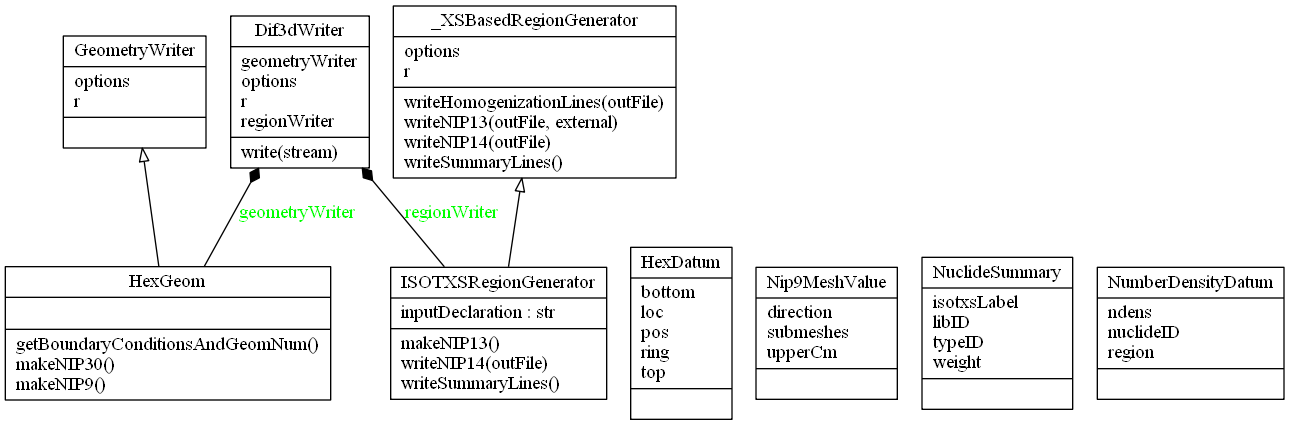
-
class
armicontrib.dif3d.inputWriters.
NuclideSummary
(libID: str, typeID: str, weight: float, isotxsLabel: str)[source]¶ Bases:
NamedTuple
An entry for single nuclide in A.SUMMAR
Create new instance of NuclideSummary(libID, typeID, weight, isotxsLabel)
-
class
armicontrib.dif3d.inputWriters.
Nip9MeshValue
(direction: str, submeshes: int, upperCm: float)[source]¶ Bases:
NamedTuple
An entry in a NIP card 09 mesh definition
Create new instance of Nip9MeshValue(direction, submeshes, upperCm)
-
class
armicontrib.dif3d.inputWriters.
NumberDensityDatum
(region: str, nuclideID: str, ndens: float)[source]¶ Bases:
NamedTuple
An entry in a NIP card 13 number density definition
Create new instance of NumberDensityDatum(region, nuclideID, ndens)
-
class
armicontrib.dif3d.inputWriters.
HexDatum
(loc: str, ring: int, pos: int, bottom: float, top: float)[source]¶ Bases:
NamedTuple
A single hexagon definition for NIP3 card 30 in hex case
Create new instance of HexDatum(loc, ring, pos, bottom, top)
-
class
armicontrib.dif3d.inputWriters.
Dif3dWriter
(reactor, options)[source]¶ Bases:
object
Write DIF3D input file.
Uses the ARMI state plus a template.
Geometry code is handled through a GeometryWriter subclass, which allows geometric specialization through composition instead of inheritance.
Build the writer
-
class
armicontrib.dif3d.inputWriters.
GeometryWriter
(reactor, options)[source]¶ Bases:
object
Handles geometry-specific information on A.NIP3.
-
class
armicontrib.dif3d.inputWriters.
HexGeom
(reactor, options)[source]¶ Bases:
armicontrib.dif3d.inputWriters.GeometryWriter
Specialization for hexagonal geometry.
-
class
armicontrib.dif3d.inputWriters.
ISOTXSRegionGenerator
(reactor, options)[source]¶ Bases:
armicontrib.dif3d.inputWriters._XSBasedRegionGenerator
Writes number density sections of DIF3D input file when an
ISOTXS
library is used.- Parameters
cs (
Settings
) – Settings that determine what is writtenr (
Reactor
) – Reactor that this interface to which this interface is attached
Notes
ISOTXS
specific changesDATASET=ISOTXS
UNFORM=A.HMG4C
homogenization blockUNFORM=A.SUMMAR
nuclide summary block13 cards - nuclide number densities for each block
14 cards - list both the primary and secondary compositions for each block
-
makeNIP13
()[source]¶ Make the NIP card 13, which defines the isotopic compositions of all blocks.
All possible nuclides should be listed with proper XS library identifier.
- Raises
ValueError – Empty list of number densities.